In recent years, Telegram has become one of the most widely used messaging platforms. But its appeal doesn’t stop at being just a messaging app – it has grown into a powerful ecosystem that supports bots, channels, and even mini apps. These mini apps offer developers the ability to create integrated, user-friendly tools directly within Telegram without needing to switch to other apps.
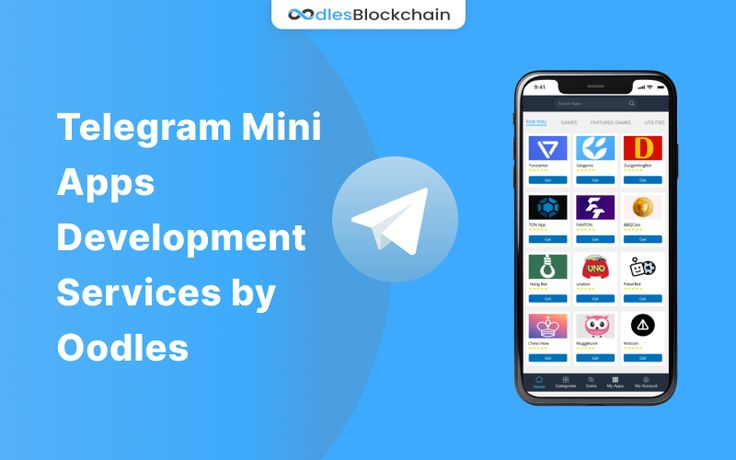
If you’ve ever wondered how to create your very own Telegram Mini App, you’re in the right place create telegram mini app! This guide will walk you through every step, from setting up the development environment to deploying your app and engaging users. Whether you’re a seasoned developer or just starting, we’ve got you covered.
What is a Telegram Mini App?
A Telegram Mini App is essentially a web app embedded directly within the Telegram interface, allowing users to interact with the app without leaving the Telegram platform. These apps can be used for a wide variety of purposes, such as games, utilities, shopping, social networking, and more.
Mini apps are developed using Telegram’s Web Apps feature and are built using regular web technologies like HTML, CSS, and JavaScript. They are designed to run in a secure, isolated environment with easy integration with Telegram’s core features.
Prerequisites
Before you dive in, make sure you have:
- Basic knowledge of web development (HTML, CSS, JavaScript)
- A Telegram account
- A Telegram bot (which you can create through @BotFather)
If you haven’t created a bot yet, here’s how to do it:
- Search for BotFather in Telegram.
- Start a chat with BotFather and type /newbot.
- Follow the instructions to name your bot and generate the API token.
- Store the token – you’ll need it later!
Step 1: Set Up Your Development Environment
You can develop a Telegram Mini App using any web development environment you’re comfortable with. A simple text editor like VS Code or Sublime Text works well.
- Make sure you have an HTTP server to serve your app. For local development, you can use simple servers like Live Server (VS Code extension) or set up a local server using Node.js.
- For deployment, you’ll need a hosting provider like Heroku, Netlify, or Vercel. These platforms offer simple deployment options for web apps.
Step 2: Register Your Mini App
To make your app available within Telegram, you’ll need to register it in the Telegram Bot API:
- In the BotFather chat, use the command /setwebapp to configure the Mini App URL for your bot.
- Enter the URL of your web app (it can be a test URL for now).
- This URL will serve as the entry point for your app when users interact with the bot.
Step 3: Build the Web App
Now, let’s start building the Mini App. For the purpose of this guide, we will create a simple “Hello, World!” web app. Follow these steps:
- Create a new HTML file (index.html):
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Telegram Mini App</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
padding: 50px;
}
</style>
</head>
<body>
<h1>Hello, Telegram User!</h1>
<button id=”sendMessageButton”>Send Message to Bot</button>
<script>
// This function allows you to interact with Telegram’s Web App API
function sendMessageToBot() {
if (window.Telegram.WebApp) {
Telegram.WebApp.sendData(“Hello from the Mini App!”);
}
}
document.getElementById(“sendMessageButton”).addEventListener(“click”, sendMessageToBot);
</script>
</body>
</html>
- Explanation:
- This HTML page contains a simple button that sends a message back to the bot.
- The Telegram.WebApp.sendData() function is used to send data from the mini app to the Telegram bot.
- Customize Your App:
- You can build on this foundation by adding more interactive elements, like forms, games, shopping carts, etc.
- You can use JavaScript to interact with Telegram’s deep integration, such as accessing the user’s name or sending callback queries.
Step 4: Set Up Communication Between Your App and Telegram Bot
To allow your mini app to interact with Telegram, you need to handle the data sent from the app back to the bot.
- Set up an endpoint (e.g., using Node.js or PHP) that receives data sent from your mini app.
- Use Telegram’s Bot API to send messages back to the user or perform actions based on the data received.
Here’s a simple Node.js example using express to handle data from the mini app:
const express = require(‘express’);
const axios = require(‘axios’);
const app = express();
const TELEGRAM_API_TOKEN = ‘YOUR_BOT_API_TOKEN’;
const TELEGRAM_CHAT_ID = ‘YOUR_CHAT_ID’;
app.use(express.json());
app.post(‘/webapp-data’, async (req, res) => {
const message = req.body.data;
// Send the received data to the Telegram chat
try {
await axios.post(`https://api.telegram.org/bot${TELEGRAM_API_TOKEN}/sendMessage`, {
chat_id: TELEGRAM_CHAT_ID,
text: `Received data: ${message}`,
});
res.status(200).send(‘Message sent’);
} catch (error) {
res.status(500).send(‘Error sending message’);
}
});
app.listen(3000, () => console.log(‘Server is running on http://localhost:3000’));
Step 5: Deploy Your App
Once your mini app is ready, it’s time to deploy it. Here’s how you can do it with a service like Vercel or Netlify:
- Vercel: Create an account and connect your GitHub repository, then deploy the app. Vercel will automatically detect your project and deploy it.
- Netlify: You can also connect a GitHub repo or upload your project files directly.
Once deployed, use the deployed URL to configure your bot’s web app URL in BotFather.
Step 6: Test the Mini App
After deploying, test your mini app by:
- Opening the Telegram bot on your phone or desktop.
- Starting a conversation with your bot and triggering the mini app.
- Interacting with the mini app and verifying that it works correctly.
Step 7: Engage Users and Improve the App
Now that your app is live, consider adding features such as:
- User authentication (e.g., sign-in via Telegram).
- Handling user input (forms, text input).
- Real-time interactions like notifications or updates.
Conclusion
Creating a Telegram Mini App can be a fun and rewarding project that allows you to leverage the powerful Telegram platform for building interactive apps. Whether you’re building a game, utility, or a business tool, this guide should give you the essential steps to get started. Happy coding!
This post provides an overview, but be sure to explore the official Telegram Bot API documentation for more advanced features and functionalities.